Microsoft.visualstudio.testtools.unittesting Mac
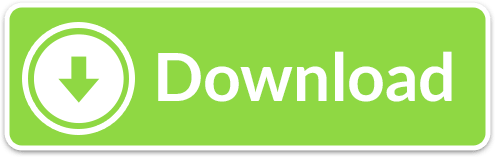
-->
Definition
2012-8-13 Can't find the reference 'Microsoft.VisualStudio.TestTools.UnitTesting;'? How to solve this problem? Hi Journey31, I feel you could reference this link named Where is the Microsoft.VisualStudio.TestTools.UnitTesting namespace on VS2010? You need to add this reference inside your project,It's called Microsoft.VisualStudio. Microsoft.VisualStudio.QualityTools.UnitTestFramework.dll Visual Studio Express では単体テストを使用できないことに注意してください。 私はC# visual studio project次のようなエラーが発生しています。 The type or namespace name 'VisualStudio' does not exist in. Use the MSTest framework in unit tests. The MSTest framework supports unit testing in Visual Studio. Use the classes and members in the xref:Microsoft.VisualStudio.TestTools.UnitTesting namespace when you are coding unit tests. How to get Microsoft.VisualStudio.QualityTools.UnitTestFramework and related dlls via VS2017 Build Tools? Visual Studio 2017 version 15.2 unit-test KY Lee reported Jun 22, 2017 at 09:34 AM.
Overloads
Fail() | Throws an AssertFailedException. |
Fail(String) | Throws an AssertFailedException. |
Fail(String, Object) | Throws an AssertFailedException. |
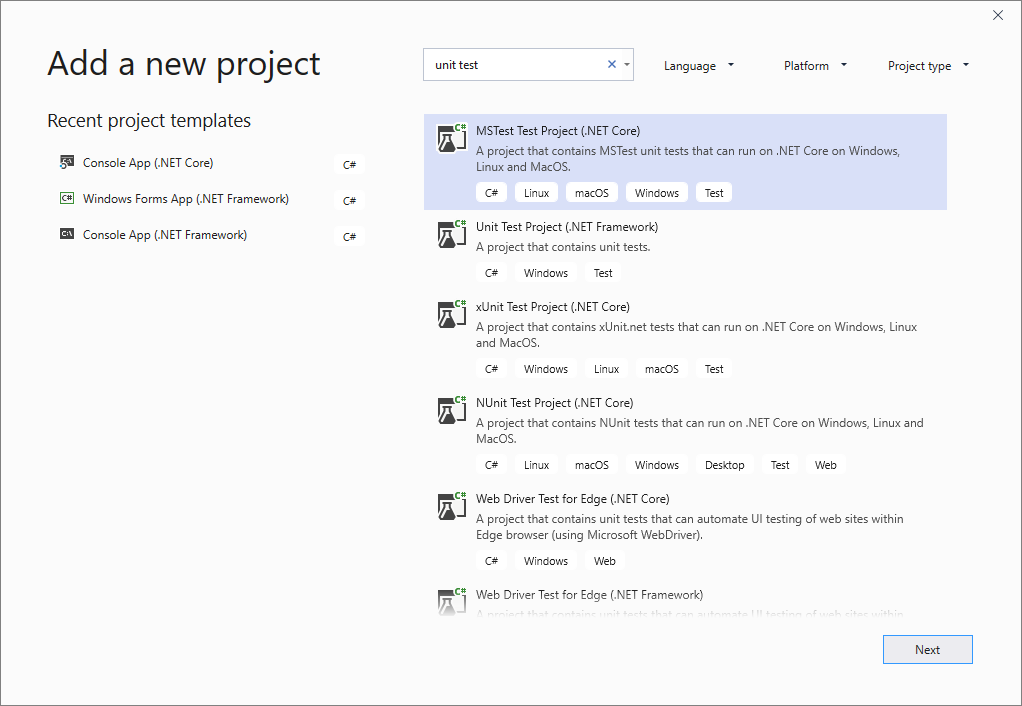
Exceptions
Always thrown.
Parameters
- message
- String
The message to include in the exception. The message is shown intest results.
Exceptions
Always thrown.
Parameters
- message
- String
The message to include in the exception. The message is shown intest results.
- parameters
- Object
An array of parameters to use when formatting message
.
Exceptions
Always thrown.
Applies to
title | ms.date | ms.topic | ms.author | author | manager | ms.workload |
---|---|---|---|---|---|---|
09/27/2019 | mikejo | jillfra |
This article describes one way to create unit tests for a C# class in a UWP app.
The Rooter class, which is the class under test, implements a function that calculates an estimate of the square root of a given number.
This article demonstrates test-driven development. In this approach, you first write a test that verifies a specific behavior in the system that you're testing, and then you write the code that passes the test.
Create the solution and the unit test project
On the File menu, choose New > Project.
Search for and select the Blank App (Universal Windows) project template.
Name the project Maths.
In Solution Explorer, right-click on the solution and choose Add > New Project.
Search for and select the Unit Test App (Universal Windows) project template.
Name the test project RooterTests.
Verify that the tests run in Test Explorer
Insert some test code into TestMethod1 in the UnitTest.cs file:
The xref:Microsoft.VisualStudio.TestTools.UnitTesting.Assert class provides several static methods that you can use to verify results in test methods.
::: moniker range='vs-2017'
Microsoft.visualstudio.testtools.unittesting Mac Pro
- On the Test menu, choose Run > All Tests.
::: moniker-end
::: moniker range='>=vs-2019'
- On the Test menu, choose Run All Tests.
::: moniker-end
The test project builds and runs. Be patient because it may take a little while. The Test Explorer window appears, and the test is listed under Passed Tests. The Summary pane at the bottom of the window provides additional details about the selected test.
Add the Rooter class to the Maths project
In Solution Explorer, right-click on the Maths project, and then choose Add > Class.
Name the class file Rooter.cs.
Add the following code to the Rooter class Rooter.cs file:
The Rooter class declares a constructor and the SquareRoot estimator method. The SquareRoot method is only a minimal implementation, just enough to test the basic structure of the testing setup.
Add the
public
keyword to the Rooter class declaration, so the test code can access it.
Add a project reference
Add a reference from the RooterTests project to the Maths app.
In Solution Explorer, right-click on the RooterTests project, and then choose Add > Reference.
In the Add Reference - RooterTests dialog box, expand Solution and choose Projects. Select the Maths project.
Add a
using
statement to the UnitTest.cs file:Open UnitTest.cs.
Add this code below the
using Microsoft.VisualStudio.TestTools.UnitTesting;
line:
Add a test that uses the Rooter function. Add the following code to UnitTest.cs:
The new test appears in Test Explorer in the Not Run Tests node.
To avoid a 'Payload contains two or more files with the same destination path' error, in Solution Explorer, expand the Properties node under the Maths project, and then delete the Default.rd.xml file.
::: moniker range='vs-2017'
In Test Explorer, choose Run All.
The solution builds and the tests run and pass.
::: moniker-end
::: moniker range='>=vs-2019'
In Test Explorer, choose Run All Tests.
The solution builds and the tests run and pass.
::: moniker-end
You've set up the test and app projects and verified that you can run tests that call functions in the app project. Now you can begin to write real tests and code.
Iteratively augment the tests and make them pass
Add a new test called RangeTest:
[!TIP]We recommend that you do not change tests that have passed. Add a new test instead.
Run the RangeTest test and verify that it fails.
[!TIP]Immediately after you write a test, run it to verify that it fails. This helps you avoid the easy mistake of writing a test that never fails.
Enhance the code under test so that the new test passes. Change the SquareRoot function in Rooter.cs to this:
::: moniker range='vs-2017'
- In Test Explorer, choose Run All.
::: moniker-end
::: moniker range='>=vs-2019'
- In Test Explorer, choose Run All Tests.
::: moniker-end
All three tests now pass.
[!TIP]Develop code by adding tests one at a time. Make sure that all the tests pass after each iteration.
Refactor the code
In this section, you refactor both app and test code, then rerun tests to make sure they still pass.
Simplify the square root estimation
Simplify the central calculation in the SquareRoot function by changing one line of code, as follows:
Run all tests to make sure that you haven't introduced a regression. They should all pass.
[!TIP]A stable set of good unit tests gives confidence that you have not introduced bugs when you change the code.
Eliminate duplicated code
The RangeTest method hard codes the denominator of the tolerance variable that's passed to the xref:Microsoft.VisualStudio.TestTools.UnitTesting.Assert method. If you plan to add additional tests that use the same tolerance calculation, the use of a hard-coded value in multiple locations makes the code harder to maintain.
Add a private helper method to the UnitTest1 class to calculate the tolerance value, and then call that method from RangeTest.
Run RangeTest to make sure that it still passes.
[!TIP]If you add a helper method to a test class, and you don't want it to appear in Test Explorer, don't add the xref:Microsoft.VisualStudio.TestTools.UnitTesting.TestMethodAttribute attribute to the method.
See also
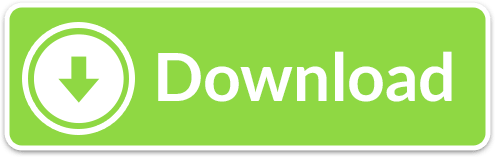